The DHT20 is a high-precision temperature and humidity sensor that communicates using the I2C protocol. This sensor offers improved accuracy and reliability compared to its predecessors, with a humidity measurement range of 0-100% RH (±3% accuracy) and a temperature range of -40 to 80°C (±0.5°C accuracy). The sensor is particularly suitable for environmental monitoring, HVAC systems, and IoT applications.
Pinout
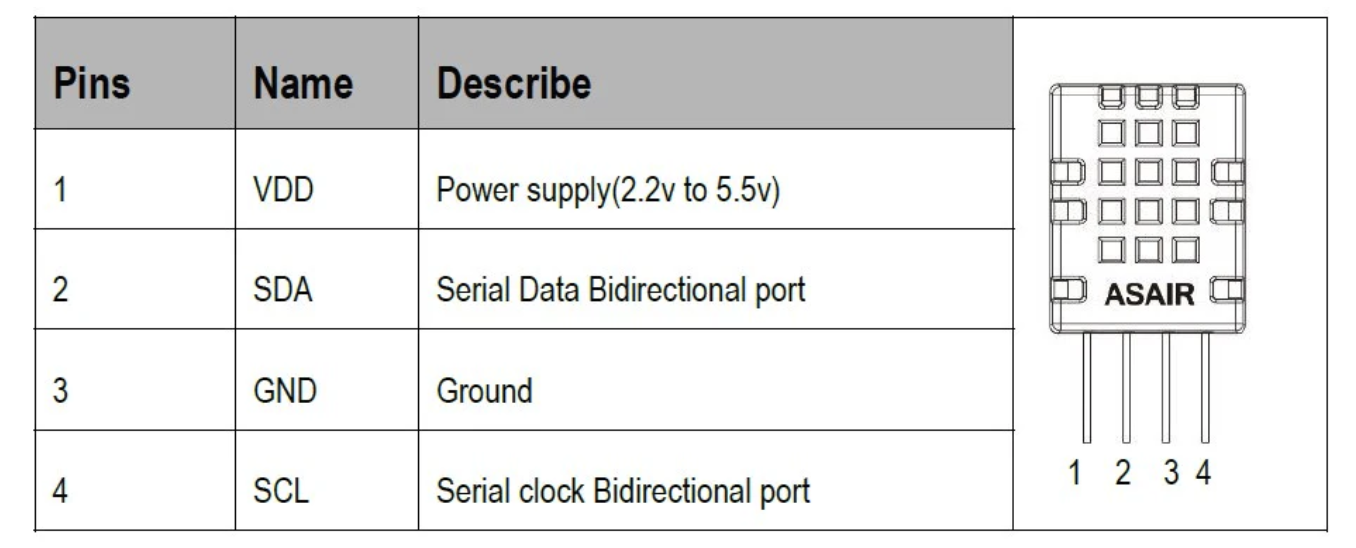
I2C Address
Before using the code, run the I2C scanner to verify and obtain the correct I2C address for your DHT20 sensor. By default, the I2C address of the DHT20 is 0x38.
I2C Address Scan
Wiring
DHT20 | Arduino |
---|---|
VDD | 5V |
GND | Ground |
SDA | SDA |
SCL | SCL |
Library
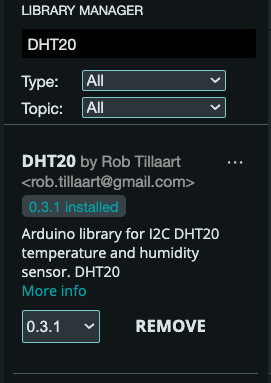
Code
#include "DHT20.h"
DHT20 DHT;
void setup() {
Serial.begin(9600);
Wire.begin();
DHT.begin();
}
void loop() {
if (DHT.read() == DHT20_OK) {
Serial.print("Humidity (%): ");
Serial.print(DHT.getHumidity(), 1);
Serial.print(" , ");
Serial.print("Temp (°C): ");
Serial.println(DHT.getTemperature(), 1);
} else {
Serial.println("DHT Sesnor Reading Error");
}
delay(5000);
}
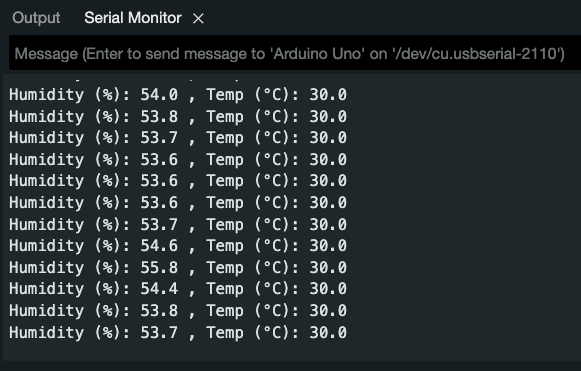
Summary
This example demonstrates basic usage of the DHT20 sensor with an Arduino board. The code initializes the I2C communication, reads temperature and humidity values every 5 seconds, and outputs the readings to the Serial Monitor. The DHT20 library handles all the low-level communication and data conversion, making it straightforward to integrate this sensor into your projects.