Table of Contents
Here’s a simple ESP32 Bluetooth Classic example that demonstrates how to set up an ESP32 as a Bluetooth Classic Serial Server. This example uses the Bluetooth Serial library to communicate with a Bluetooth Classic client (e.g., a smartphone or PC).
Code for ESP32 (Bluetooth Classic Serial Server)
Explanation:
- BluetoothSerial: The BluetoothSerial class is used to create a Bluetooth Classic serial connection.
- SerialBT.begin(“Lonely_Binary_ESP32”): This initializes the Bluetooth module with a name (ESP32_BT_Server) that will be used by clients to identify the device.
- SerialBT.available(): This checks if data is available from the connected Bluetooth client.
- SerialBT.read(): Reads data from the Bluetooth client and prints it to the Serial Monitor.
- SerialBT.println(): Sends a message to the Bluetooth client. In this case, the ESP32 will send “Hello from ESP32 Bluetooth server!” every second.
How to Test:
- Upload the code to your ESP32.
- Enable Bluetooth on your PC and search for Bluetooth devices.
- You should see Lonely_Binary_ESP32 in the list of available devices. Pair with it.
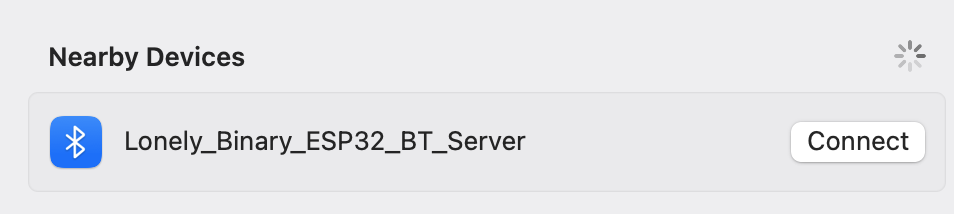
- Use a Bluetooth Terminal app for Android or a Termius App on your PC to connect to the ESP32.
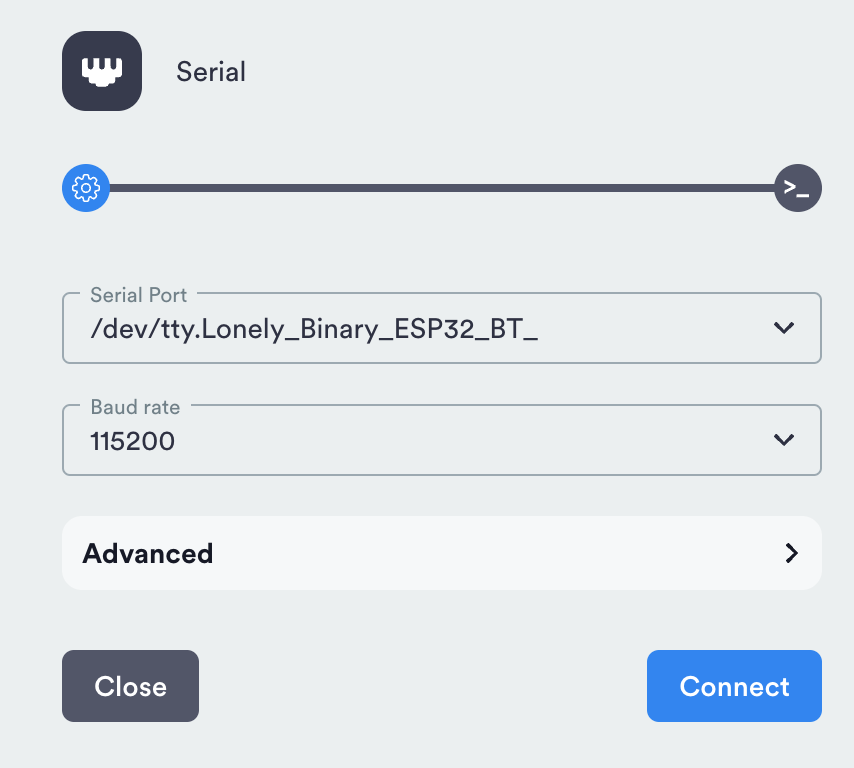
- Once connected, the app should display messages like “Hello from ESP32 Bluetooth server!” from the ESP32.
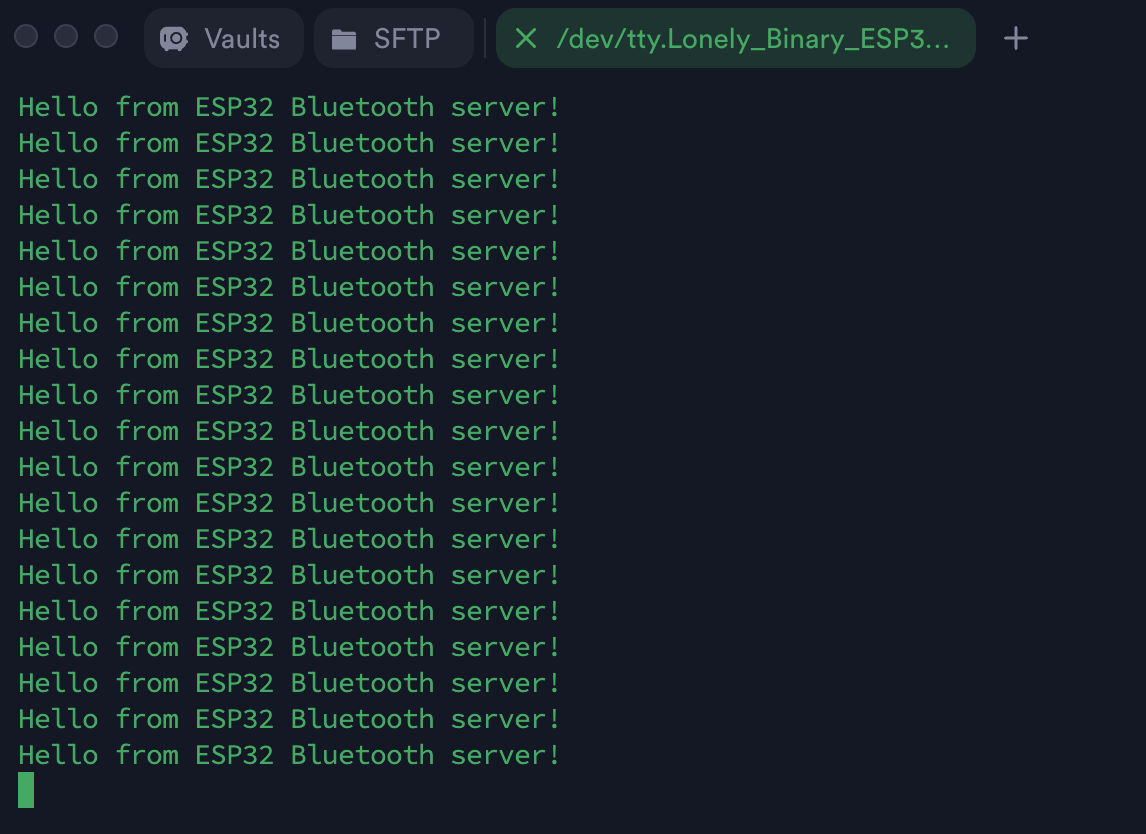
- You can also send data from the app to the ESP32, and it will be printed in the Serial Monitor.