Table of Contents
This example will set up the ESP32 as a BLE server with a writable characteristic. The value written to the characteristic will be printed in the serial monitor.
Code for ESP32 (Server):
Explanation:
- The ESP32 is initialized as a BLE server with a custom service and a characteristic (READ and WRITE properties).
- The client can write a new value to this characteristic, and the server will print the new value in the Serial Monitor.
- The server advertises itself so that a BLE client can discover and connect to it.
Client Example (Using a Mobile App like nRF Connect):
- Open a BLE client app (such as nRF Connect on Android or iOS).
- Scan for nearby BLE devices and find the ESP32_BLE_Server.
- Connect to the ESP32 BLE server.
- Locate the writable characteristic (87654321-4321-4321-4321-abcdef123456).
- Write a new value (e.g., “Hello from client”) to the characteristic.
- The ESP32 BLE server will print the received value on the Serial Monitor.
Testing:
When you write a value to the characteristic (e.g., “Lonely Binary”), the ESP32 server will print:
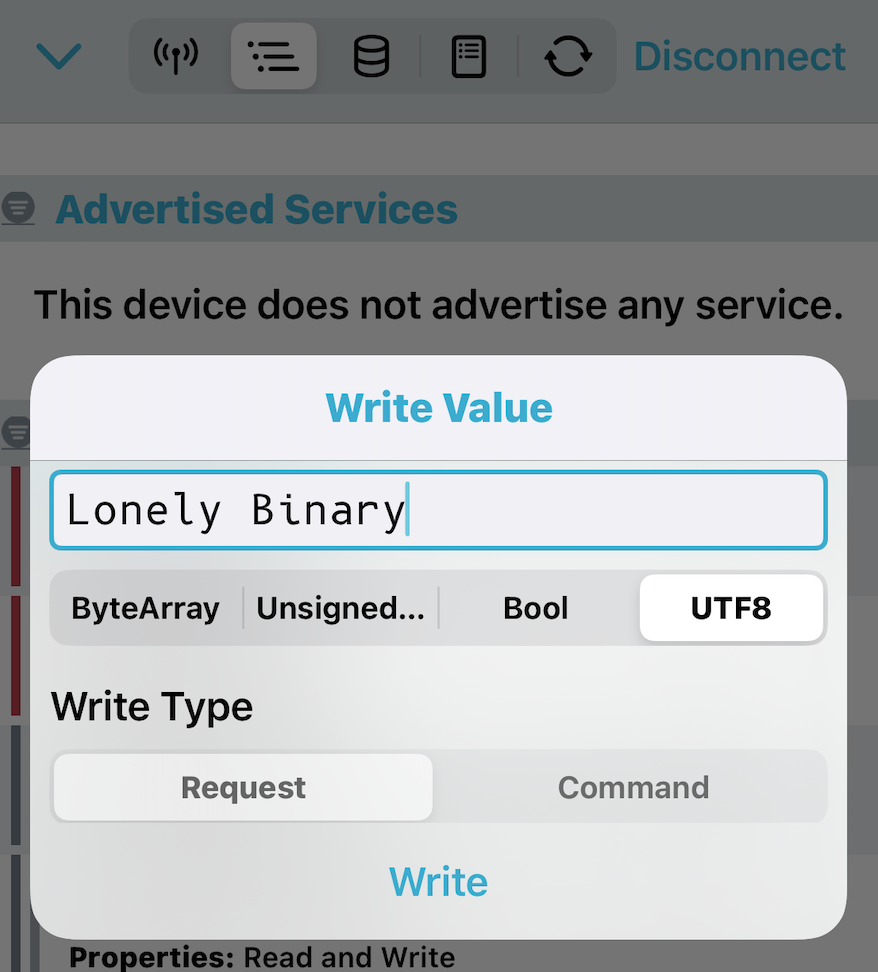
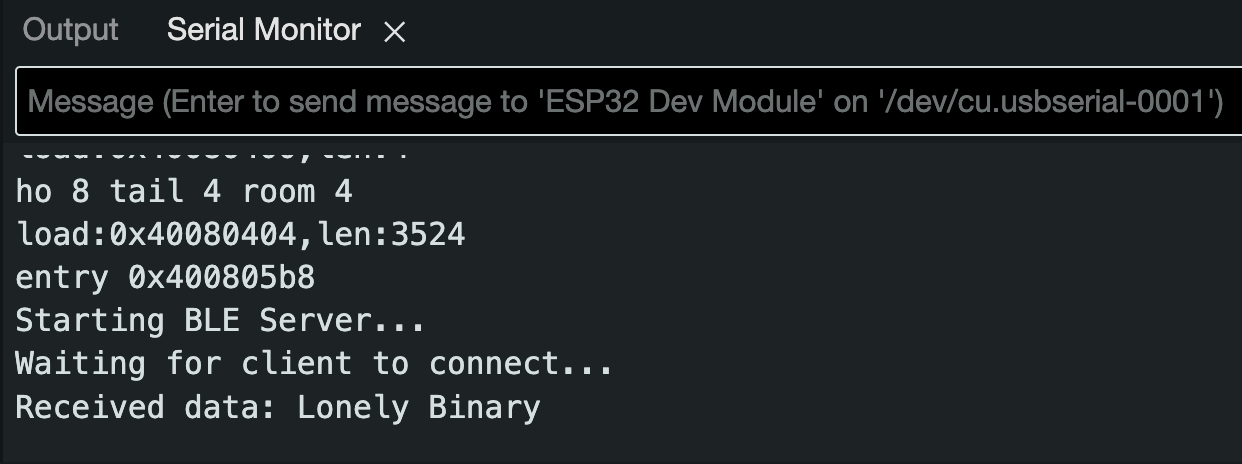